It’s no secret that testing ensures the quality, reliability, and functionality of software products such as modern web apps. If well-organized, it contributes to delivering a seamless user experience, meeting customer expectations, and building trust in the software being developed.
However, as their projects grow in complexity, teams face some challenges associated with managing and executing tests in test automation. Such factors as increased codebase size, diverse testing scenarios, long test execution times, etc. lead to potential inefficiencies in test cases scenarios, inability to maintain a clear understanding of test coverage, slowing down the overall testing and development process.
This is precisely where the significance of the Playwright testing tool emerges in test automation. The adoption of Playwright testing framework coupled with a focus on effective test organization helps teams deliver high-quality software in an efficient manner. With its rich set of features, Playwright empowers developers and testers to script complex testing scenarios, simulate user interactions, provide cross browser testing and verify the functionality of software products thoroughly.
Key reasons to group and organize tests in Playwright
Organizing Playwright tests in the testing framework is crucial for several reasons and contributes to maintainability, collaboration, and efficiency throughout the development and test automation process. Let’s take a look at why group and organize Playwright tests may be worthwhile for your team.
- Clarity and readability. In fact, test suites expand when performing end-to-end testing for modern web apps. That’s why maintaining a clear and readable test structure becomes imperative. If automated tests are well organized, it provides a roadmap of test functionalities and improves team collaboration.
- Achieving test isolation and independence. By grouping tests, you can isolate different functionalities or modules and deliver full test isolation with no overhead. This allows teams to run them individually or in specific groups as well as let them remain independent.
- Easy debugging. With well-organized tests, debugging becomes more straightforward. If a test failure occurs, you can quickly identify the relevant group and narrow down the issue. This significantly reduces the time required to fix problems.
- Maintainability. With well-organized Playwright tests, you can maintain them with ease. This not only helps you simplify the process of identifying and updating test cases when application changes occur, but you can also reduce the risk of introducing errors during maintenance.
- Efficient test execution. With strategic test organization, you can run tests efficiently. When running specific sets of tests, you can reduce the overall time required for execution and get quick feedback loops.
How to organize and group tests in Playwright framework
From grouping tests based on features and modules to leveraging advanced techniques like the Page Object Model (POM), we will delve into various approaches that empower your testing efforts and help you improve your automation testing skills as well as run the first effective Playwright test script.
#1: Organize by priority, feature or module
Grouping tests by feature or module is known as a fundamental approach that maintains logical structure. This allows developers to create test groups with a focus on a specific functionality or module. They can prioritize Playwright test or tests accordingly and run critical tests to ensure they are executed first. Thus, it allows them to improve clarity and locate relevant Playwright test or tests easier. Here is an example folder structure:
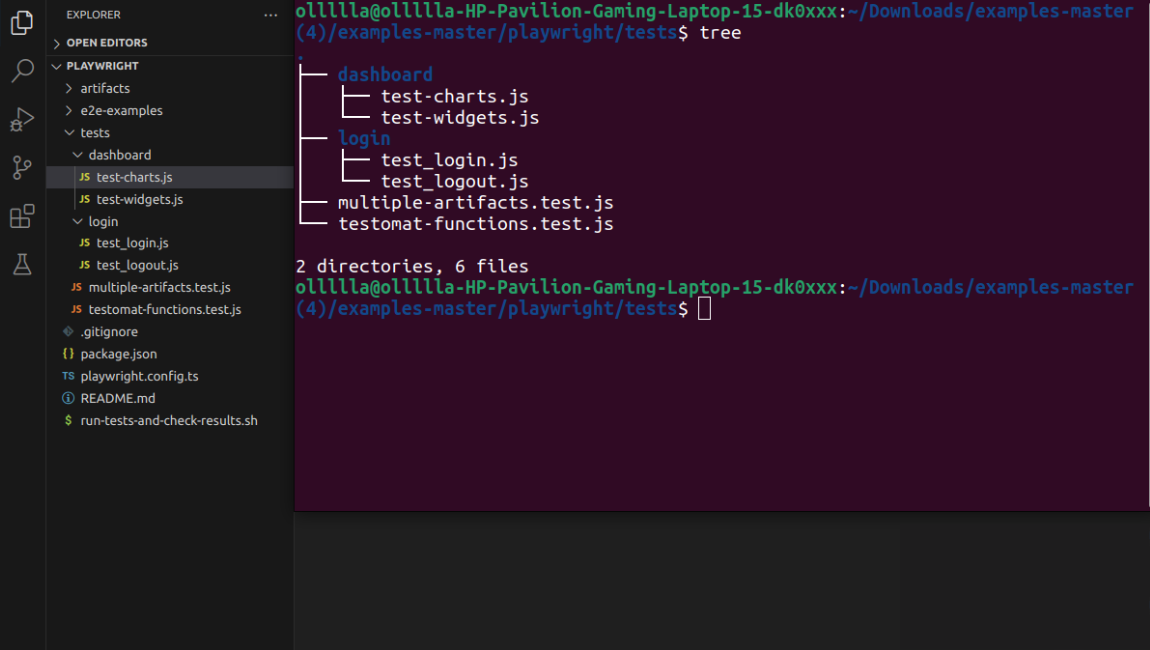
In this example, tests related to the login functionality are grouped together in the ‘login’ directory, and tests for the dashboard feature are in the ‘dashboard’ directory. This makes it easy to identify and run tests for specific areas of the web application testing.
#2: Using Test Suites
Applying test suites provides a higher-level organization of tests. By grouping tests into suites based on Playwright testing scenarios, such as smoke tests, regression tests, or end-to-end tests you can perform any testing type based on your testing needs.
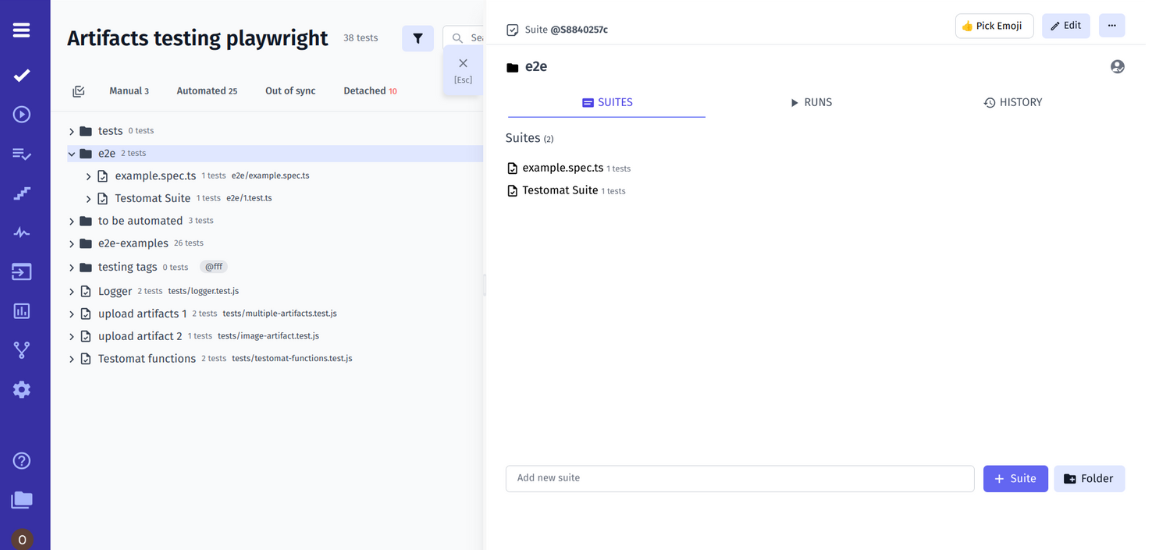
Key Components of a Test Suite in Playwright:
- Descriptive Naming. Give your test suite a descriptive and meaningful name that describes the purpose or focus of the tests it contains. For example, a Playwright test suite named “Product Checkout” might encompass all tests related to the checkout process.
- Nested Test Cases. Within a test suite, you can nest individual test cases using the test function. Nesting allows for a hierarchical structure, enhancing readability and organization.
- Before and After Hooks. In your Playwright framework, tests can be executed as suites, if they are completed with before and after hooks. These hooks enable you to perform setup and teardown operations specifically for the entire suite, ensuring a consistent state before and after test execution. It allows avoid the parallel testing initially implemented in Playwrright. Follow Playwright Docs
import { test, expect } from '@playwright/test';
test.describe('two tests', () => {
test('one', async ({ page }) => {
// ...
});
test('two', async ({ page }) => {
// ...
});
});
When using test suites in Playwright, teams can derive the following benefits:
- Teams easier to understand the structure of your test suite thanks to a logical and hierarchical organization of tests.
- Teams can selectively execute specific suites based on their test automation needs.
- Teams can perform efficient maintenance in terms of changes made to the suite level that are automatically reflected in all nested tests.
- As your project evolves, test suites scale seamlessly. New tests can be added to existing suites, and new suites can be introduced to accommodate additional functionalities.
#3: Parametrized Test Scenarios
Incorporating parametrized tests eliminates the need to write tests separately for similar scenarios. This helps you use parameters to dynamically execute the same test with different inputs.
const {
test,
expect
} = require('@playwright/test');
const validCredentials = [{
username: 'user1',
password: 'pass123'
},
{
username: 'user2',
password: 'secure456'
},
// Additional sets of credentials
];
test('Login with Valid Credentials', async ({
page
}, testData) => {
const {
username,
password
} = testData;
await page.goto('https://example.com/login');
await page.fill('#username', username);
await page.fill('#password', password);
await page.click('#loginButton');
// Assertion and further test steps
}).each(validCredentials);
With parameterization, you can eliminate redundancy: the steps are written once, and the test is executed for each set of credentials. In addition to that, new sets of credentials can be easily added to the validCredentials
array without duplicating test logic. Any changes to the login process only need to be made in one place that improves maintainability.
That’s why applying parameterization allows teams to create more efficient and maintainable Playwright tests, especially when dealing with scenarios that involve multiple input variations. This approach contributes to a scalable and readable test suite and speeds up test automation process.
By the way, the Test Management system testomat.io is a modern solution that supports the feature of displaying parameterized tests.
#4: Tagging Tests
When it comes to tagging tests, you can take into account attributes like priority, type, or environment to organize flexible categorization. With tags in place, you can selectively execute and provide an easy way to filter tests for specific scenarios. Let’s consider a test suite for an e-commerce website where we want to tag Playwright tests based on their priority and run only a subset of tests using these tags.
const {
test,
expect
} = require('@playwright/test');
// Test with a high priority
test.describe('Product Checkout @highPriority', () => {
test('Add Product to Cart', async ({
page
}) => {
// Test steps for adding a product to the cart
});
test('Proceed to Checkout', async ({
page
}) => {
// Test steps for proceeding to checkout
});
// Additional tests related to high-priority checkout
});
// Test with a medium priority
test.describe('Product Reviews @mediumPriority', () => {
test('View Product Reviews', async ({
page
}) => {
// Test steps for viewing product reviews
});
test('Write a Product Review', async ({
page
}) => {
// Test steps for writing a product review
});
// Additional tests related to medium-priority product reviews
});
// Test with a low priority
test.describe('User Account @lowPriority', () => {
test('Login to User Account', async ({
page
}) => {
// Test steps for logging into a user account
});
test('Update User Profile', async ({
page
}) => {
// Test steps for updating user profile information
});
// Additional tests related to low-priority user account management
});
As you see, tests are organized into groups based on priority, and each group is tagged accordingly – @highPriority, @mediumPriority, @lowPriority. With the --test-match
option, teams can selectively execute tests based on specific tags that are particularly useful during different phases of development or when focusing on specific functionalities. In addition to that, they can adapt their testing strategy based on priorities.
As a result, tagging tests allow teams to selectively execute specific groups of tests, run them in different environments (e.g., development, staging, production), prioritize regression tests based on their criticality, etc.
Note: When you import Playwright tests into testmatio, the tests which are marked with your @tags, including the @tags will be recognized and imported automatically by the importer. Moreover, with reporter you can check Test Statistic by @tags
#5: Parallel Playwright Test Execution
Organize tests that can run independently into groups to take advantage of parallel execution of the browser automation tests. This optimizes resource utilization and significantly reduces overall Playwright test suite execution time.
👇 Let’s discover how to organize tests for effective parallel execution in Playwright web automation testing:
- Group Independent Tests. Identify independent tests and group them together as they can run all in one without interfering.
- Utilize Playwright Test Suites. Organize your tests into logical test suites to provide a higher-level structure to run parallel tests suites.
- Implement Before and After Hooks. Utilize ‘before’ and ‘after’ hooks at the suite level to set up and tear down common configurations. This ensures that these operations don’t disrupt other concurrently running tests.
- Parameterized Playwright Testing. Utilize parameterized tests to run the same test logic with different inputs at the same time. This is especially useful when testing multiple data sets or scenarios.
- Tagging for Parallel Execution. Tag tests based on characteristics such as priority or type to selectively run specific groups of all the tests together.
- Parallel Execution Configuration. When running tests, ensure that your test runner is configured to enable parallel execution.
- Monitor Resource Usage. Keep on track the resource usage, especially when running tests in parallel and make sure that your testing environment has sufficient resources to accommodate the specified number of parallel tests.
Playwright’s Best Practices guide should help you write stable and more resilient tests.
#6: Page Object Model (POM)
Implementing the Page Object Model (POM) strategy helps teams organize tests around web pages. This design pattern promotes code reusability, maintainability, and a clear separation between test logic and page interactions. Below is an overview the key components of Page Object Model:
- Page Classes. Each web page or component of the application is represented by a page class that encapsulates all the elements, actions, etc. related to that specific page.
- Element Locators. Locators (CSS selectors, XPath, etc.) refer to the mechanisms used to identify and interact with specific elements on a web page.
- Methods for Interactions. Methods within page classes define actions that can be performed on the associated web page, such as filling out forms, clicking buttons, or navigating between pages.
- Readability and Reusability. By encapsulating page-specific details, the Page Object Model improves code readability. Reusing the same page class across multiple tests promotes consistency and reduces redundancy.
Below you can find how this model may be useful for development and testing teams:
- With POM, teams can deliver a clear and structured code organization and make the codebase modular and easy to navigate.
- Page classes encapsulate reusable components and interactions that drive promoting reusability and eliminate code duplication.
- When the UI changes, locators are updated in the corresponding page class. This provides swift adaptation to changes and reduces the impact on existing Playwright test script.
- The Page Object Model scales seamlessly as the application grows. This helps teams maintain a scalable and organized Playwright test suite.
Recommendation checking out the following article:
Benefits Once Tests Have Been Organized And Grouped
Here we are going to explore the benefits that teams can reap once tests have been thoughtfully organized and grouped. Don’t hesitate to read!
#1: Fostered Collaboration
A well-organized test suite boosts team collaboration as team members can quickly locate and contribute to specific test groups. Software engineers and testers not only locate relevant test groups quickly but also gain insights into diverse testing scenarios. What’s more, they can also improve their skills when facing varied testing challenges.This fosters a culture of continuous learning and drives a collaborative learning environment that enhances the team’s proficiency and ultimately leads to more effective and innovative testing approaches.
#2: Streamlined Test Suite Maintenance
When tests are logically grouped, it is easy to update and modify them. This reduces the risk of introducing errors during maintenance. In addition to that, the streamlined maintenance promotes agility and helps teams adapt to changing software or client’s requirements. A well-organized test suite allows teams to identify and address specific functionalities or features that need to be modified. This agility appears to be crucial in dynamic development and testing environments where rapid changes happen. The reduced risk of errors during maintenance not only provides reliability of the testing process but also allows teams to respond promptly to changes in project priorities and contributes to overall project success.
#3: Quick Feedback Loops
Selective test run and parallel test execution lead to faster feedback loops. Teams can focus on specific test scenarios or execute tests all at once. This saves valuable time in the testing process. In addition to that, software engineers can receive prompt feedback on the changes they made and address issues early in the development cycle.
#4: Efficient Resource Management
Only by tailoring test runs to specific scenarios can teams efficiently allocate resources and make sure that testing efforts align with project priorities. This strategic use of resources improves overall testing efficiency and allows teams to dynamically adapt to project timelines and deliver quicker insights into software quality.
#5: Scalability Improvements
If a software product undergoes changes, a well-structured test suite demonstrates scalability. New tests can be seamlessly integrated, and adjustments to existing tests can be made without jeopardizing the overall structure. Thanks to the modular nature of grouped tests, teams can drive the integration of new test cases effortlessly and accommodate functionality that expands. This adaptability ensures that adjustments to existing tests made doesn’t compromise the overall structure and results in a scalable testing ecosystem.
#6: Clarity through Test Documentation
Well-organized tests can be considered as documentation units and uncover specific information about functionalities or features of the software products. This documentation not only provides insights into the overall testing process, but also fosters better understanding among the development and testing teams. Additionally, organized tests drive visibility into testing progress and quality metrics as well as help teams make informed decisions.
Ready to let your test cases play in unison with Playwright framework?
While Playwright equips developers with powerful testing capabilities, optimizing test organization is key to unlocking its full potential. Grouping and organizing tests in your Playwright framework leads to building an efficient testing infrastructure.
When teams adhere to strategies such as organizing by feature, using test suites, parametrizing tests, tagging tests, providing parallel execution, and implementing the POM approach, they can make sure that their testing efforts are streamlined and adaptable to the evolving project requirements.
Only by strategically grouping and organizing tests can teams improve test efficiency, scalability, and maintainability. If you have any doubts, contact our experts to find how we can help you.