The software development field is characterized by a high-level competition. What’s more, user requirements for the quality of created digital solutions are constantly growing. Therefore, QA and development teams must do everything possible to release premium quality software products to the market. One of the ways to achieve this goal is to launch unit testing on the project.
What is Unit Testing?
Unit testing is a special software testing technique carried out by developers to test every single unit and component of the software under test. It is the smallest form of software testing because it checks every small unit of a larger software product.
Thus, it is good to initially validate software as well as a convenient way to Devs check yourself, they can test methods and functions. So, if your developers do not write unit tests still 🤔
Why Should Your Team Consider Writing Unit Tests for JS Codebase?
JavaScript is a dynamic, object-oriented, prototype programming language whose popularity grows yearly.
For example, it has won first place among the languages used to develop web resources. And it is not surprising because its use allows you to add to websites animated objects, 3D graphics, interactive maps, and regularly updated content.
Because of this demand among developers, testers need to provide quality testing of JavaScript projects.
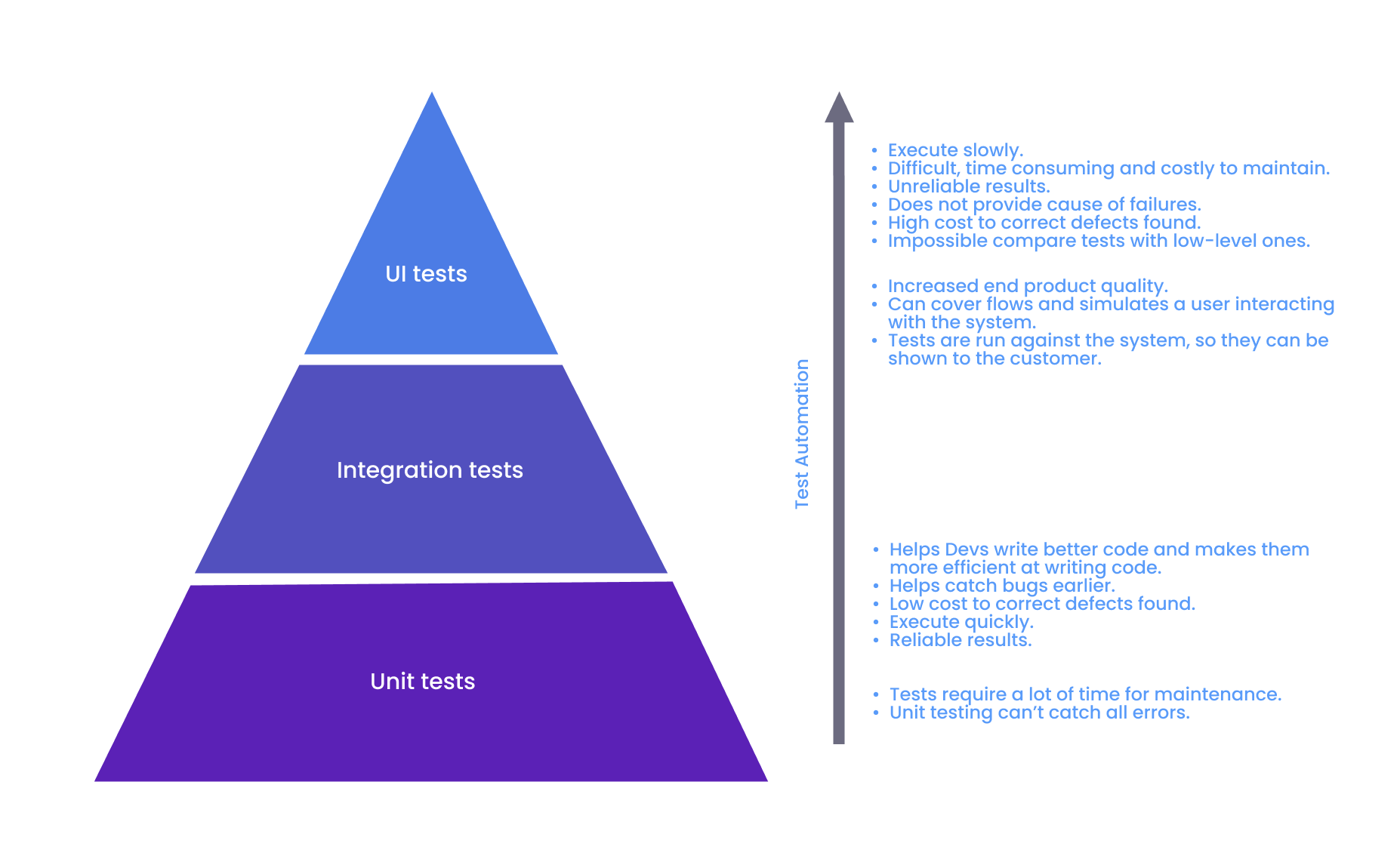
Along with this reason, the importance of JavaScript unit testing is confirmed by other benefits of the approach:
- Checking the performance of the system at the level of its independent components. Javascript developers don’t need to check all the code for bugs. They can test individual components of a program, for example, after code changes, and then merge them into a common codebase. This makes the software testing process much faster and easier.
- Improving code quality. Sometimes developers write unit test cases before they start working on the product. This method of software creation is called Test Driven Development, or TDD. The peculiarity of this approach is that the requirements for a digital solution are converted into test suites, and multiple tests are performed based on them. TDD use provides high reliability of tests because they are performed in an isolated environment.
- Quick access to project documentation. In addition to performing direct testing tasks, unit tests also serve as project documentation. This makes it easy for the development team to know what functionality a particular javascript unit is responsible for. This feature is especially relevant when a developer changes.
- Saving the budget of a project. Identifying and fixing bugs at the unit testing stage is much cheaper than testing at later stages when one defect can cause major problems throughout the system.
JavaScript unit testing is an important part of the software development lifecycle, allowing you to create high-quality digital solutions and get them to market quickly while reducing company costs.
Write Unit Tests better: A Few Recommendations for Quality Testing
How well your unit tests are written will determine whether you can detect and correct a bug in time. Below you will find some recommendations on how to write effective unit tests cases:
- Don’t write bulky JavaScript test code. Unit tests should be as simple and brief as possible. This directly affects the speed at which they can be run. If this condition is not met, testers will not be able to run tests as often as the project requires.
- Describe not only positive but also negative test cases. All testers create unit tests that test the behavior of functions when used correctly. However, it is also important to test how web applications react to incorrect user actions. Such tests are very informative. They allow you to test the functionality of a program in non-standard situations.
- Divide complex functions into small components. Bulky functions that contain too many operations are difficult to test. To simplify the testing of each variable, you should break them up into smaller components.
- Use mocking in unit tests. For instance, an app that connects to the network or other programs and makes network calls is long and challenging to test. To optimize javascript unit testing, simulate a dummy API server. This will simulate network calls and speed up the testing process. Connecting to a real network or database is better done while running other types of testing, such as integration testing.
Key benefits of JavaScript Unit Testing Automation
- Improve digital solution architecture. Since this type of testing is performed for individual code sections, a failed unit test will always indicate a fault in a particular function. This allows developers to consider the system’s functionality early in development. Later on, this will save them from rewriting the code base.
- High speed of execution. Developers try to make unit tests as simple as possible, which positively affects the speed of their execution. This allows them to achieve the main goal of unit testing – timely detection of errors in software functioning.
- Quality test coverage. Unit tests significantly increase the test automation coverage index. The possibility of a parallel launch of several unit test cases also facilitates this.
- Accuracy of results obtained. Using automated testing eliminates the human factor in the testing process, which contributes to obtaining more accurate results. Modern testing frameworks also allow you to record all the information obtained and provide their users with detailed Reports and Analytics. This allows one to check and analyze all the data again after the unit test run, and present it to stockholders.
- Saving resources. Although working with the javascript testing framework requires an initial financial investment, automated testing pays for itself later on. This is due to the same coding language for developers, early detection of errors, and the ability to work together on project development and testing teams.
Eventually, JavaScript automated unit testing allows you to ensure the program functions properly in the early stages of SDLC. If you have already opted for automated testing, all that remains is to decide on the unit testing framework that will best meet the needs of your team and project.
Best Unit Testing Frameworks to Optimize Your Workflow
Many tools in today’s software market allow you to perform automatic JavaScript unit testing. Some of them are present on the market quite a long time ago. They are very familiar to testers and thus enjoy well-deserved popularity. These frameworks include Mocha, Jasmine, and Jest.
But at the same time, you should not ignore the new players on the market like Cypress, Playwright, CodeceptJS e2e testing frameworks. Because modern, progressive tools are best adapted to the needs of Agile teams.
👉 Let’s explore the capabilities of each of the next frameworks in the area of JavaScript unit testing:
Mocha
This JavaScript testing environment was initially designed for Node.js but now supports other frameworks, including React, Vue, and Angular. It is popular with users because it helps make asynchronous testing much easier.
// Getting started with Mocha
$ npm install mocha
$ mkdir test
$ $EDITOR test/test.js # or open with your favorite editor
// In your editor:
var assert = require('assert');
describe('Array', function () {
describe('#indexOf()', function () {
it('should return -1 when the value is not present', function () {
assert.equal([1, 2, 3].indexOf(4), -1);
});
});
});
// Back in terminal
$ ./node_modules/mocha/bin/mocha
Array
#indexOf()
✓ should return -1 when the value is not present
1 passing (9ms)
Benefits of Mocha:
- Flexibility. You can easily connect the third-party libraries you need to this testing environment.
- Ability to run tests in parallel. This increases the performance of the QA team but, at the same time, makes it impossible to generate some reports. To solve this problem, the framework provides the possibility of sequential testing.
- Support to write tests as ES units. In Mocha, you can create unit tests using more than just CommonJS standards.
At the same time, Mocha has such disadvantages:
- Configuration difficulties due to the need to connect third-party reporters, libraries, etc.
- Incompatibility with some plugins.
- Poor documentation.
- Lack of support for Arbitrary transpiler.
Jasmine
This is another popular testing environment that you can use to test JavaScript code and apps written in other languages, including Jasmine libraries for Ruby and Python. Jasmine is popular among teams that practice the BDD approach to software development. Although we should note that it lost his major position in the last time.
// Getting started with Jasmine
$ npm install --save-dev jasmine
$ npx jasmine init
//Set jasmine as your test script in your package.json
"scripts": { "test": "jasmine" }
// Let us examine a simple contrived example, an add function:
const add = (a, b) => a + b;
// A primitive Jasmine test without any <a href="https://testomat.io/blog/best-open-source-testing-tools-list/">testing tools</a> could look like this:
const expectedValue = 5;
const actualValue = add(2, 3);
if (expectedValue !== actualValue) {
throw new Error(
`Wrong return value: ${actualValue}. Expected: ${expectedValue}`
);
}
//The expect function together with a matcher.
const expectedValue = 5;
const actualValue = add(2, 3);
expect(actualValue).toBe(expectedValue);
$ npm test
Good explanation and examples of how to start with JavaScript Jest Unit testing you can find in Docs of Angular front-end framework.
Advantages of the framework:
- Enough features that work out of the box. With Jasmine, you don’t need to install additional libraries or change the configuration. Because of this, the tool shows quite high speed and performance.
- Clear syntax. This makes it easier to work together on a project, which is one of the priorities of modern Agile teams.
- Integration with other tools. These include the Jest testing environment, which will help you run unit tests faster, the JavaScript test coverage tool Istanbul, etc.
The disadvantages of the Jasmine framework include:
- Compatibility problems with some browsers and frameworks; you will have to install additional plugins to work with them.
- Lack of some important functions, which are provided by other platforms. For example, mocking, unit test coverage reports, and parallel testing.
Jest
This open-source testing framework is the leader in popularity among JavaScript developers. It was created by Facebook based on Jasmine and is mostly used for testing web applications written in React and React Native.
//Let's get started
$ npm install --save-dev jest
// Write a test for a hypothetical function that adds two numbers.
// But at first, create a sum.js file:
function sum(a, b) {
return a + b;
}
module.exports = sum;
//Create a file named sum.test.js. lt should contain our actual test:
const sum = require('./sum');
test('adds 1 + 2 to equal 3', () => {
expect(sum(1, 2)).toBe(3);
});
//Add the following section to your package.json:
{
"scripts": {
"test": "jest"
}
}
//Finally run
$ npm test
PASS ./sum.test.js
✓ adds 1 + 2 to equal 3 (5ms)
Using Jest has its benefits:
- High performance. This is especially important when working on large-scale projects.
- Compatibility with various apps. This environment is often used to test the quality of digital React solutions but also supports testing Angular, Node, Vue, and other apps.
- Time simulation feature. It allows you to run tests faster.
- Quality user support. You can solve most of your problems with the help of detailed documentation. If you can’t find an answer to your question, you can turn to the extensive Jest community anytime.
Minuses of the tool:
- Slowing down the testing process because of the automatic mock.
- Difficulty learning the framework;
- Lack of support from some integrated development environments can affect the availability of essential features.
Our test management solution allows you to work with tests written with popular JavaScript unit testing frameworks mentioned above, optimize the testing process and visualize its effectiveness.
The major benefits of test management:
- Rapid import of automated test cases thanks advanced reporter.
- Projects capacity with over 2000 suites and 75 000 tests.
- CI\CD test execution.
- Parallel test execution support.
- Possibility of sync automated tests with manual tests in one place.
- Converting manual tests into automatic ones.
- Real-time reporting and analytics system.
- Run history.
- Organizing tests by @tags, labels, environments, filtering, searching capabilities.
- Support of integration with third-party tools and services, like Jira project management system, Linear, AzureDevOps etc.
- Unlimited artifacts.
If you consider TMS in the context of JavaScript unit testing, its main advantage is the possibility of importing test cases from any JavaScript testing environment you have worked with before. Conveniently, this procedure is performed in a few clicks. All tests are imported into the system in CSV/XLS format. You can start working with them immediately in Testomat.io.
Bottom Line
JavaScript unit testing is a mandatory component of the software lifecycle. To save the budget, customers tend to ignore this stage of development. However, doing so is not advisable because unit tests ensure that all errors in the JavaScript code will be detected and corrected in time. Thus, you will market a high-quality digital product faster.
Writing units test is the responsibility of the developer. They can write JavaScript test code just like they write regular application source code.
To streamline the unit testing development team, it is important to choose a JavaScript unit testing framework that best meets the project’s needs. Among existing software, it is worth paying attention to multifunctional platforms that follow Agile principles and support integration with third-party tools highlighting the versatility of JavaScript unit testing frameworks.
If you still have questions? Read the other materials on the site or contact our experts, who will gladly help you!