Various testing types are applied to assess the quality of web programs, each playing a crucial role in the software development life cycle. Among them are API testing, End-to-End tests, and component testing. This material will focus on the importance of the latter testing type, specifically in creating quality web applications using Cypress testing framework.
Why Component Testing?
Before discussing why component tests are crucial in the comprehensive process of testing a web application, it’s essential to understand what this testing type entails 🤔
Component testing assesses the quality of each individual component of a digital product without their mutual integration.
Architecturally, such testing is often equated to unit or module testing, which also involves checking the functionality of individual application parts. However, there are differences between these testing types!
The main distinction between component, unit, module testing lies that in component testing operating on a “black-box” principle, meaning specialized testing engineers know nothing about the internal workings of the digital solution. In contrast, unit testing represents “white-box” testing, where the tester knows how the application functions. This line between Black Box Testing and White Box Testing as difference of Unit and Component testing you can see with the illustration below.
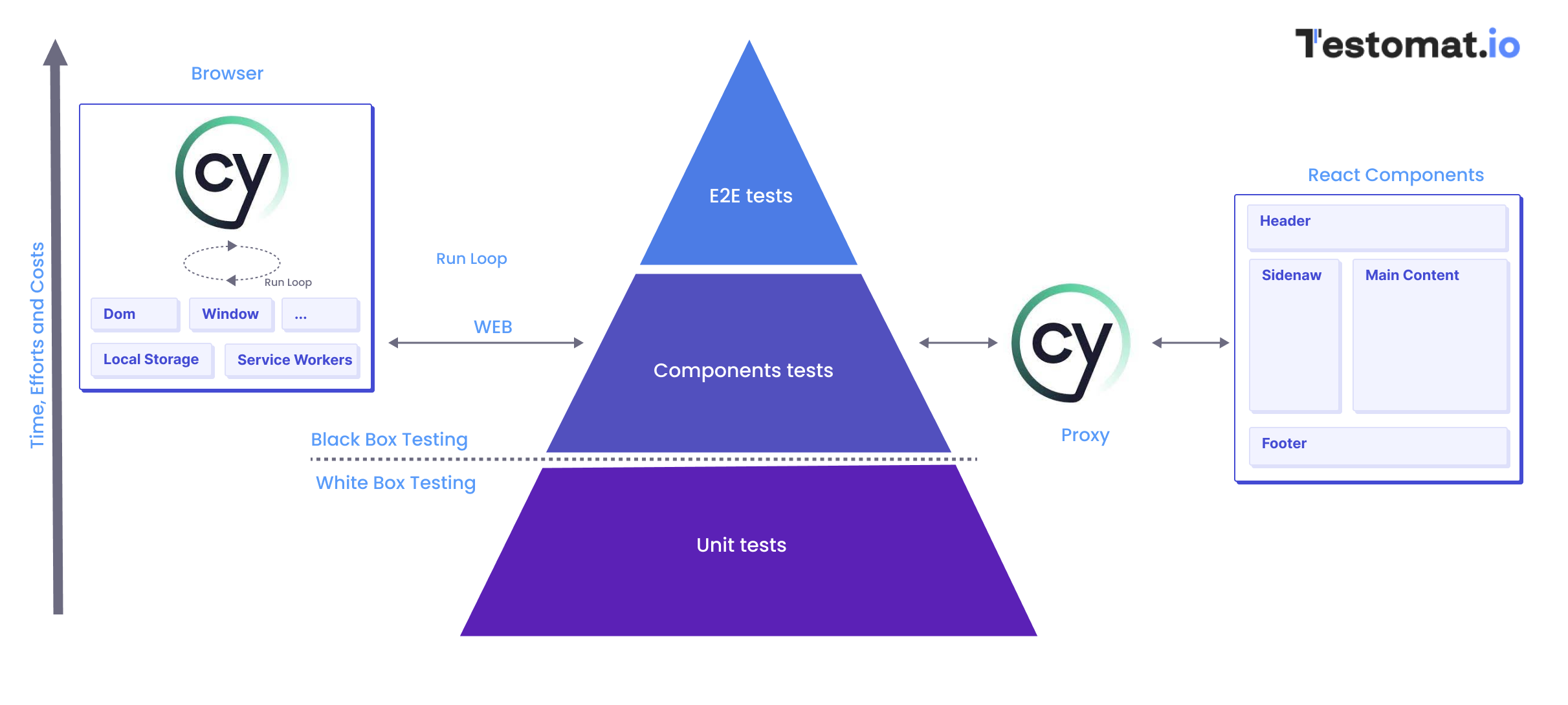
The components of a software product can be any of its elements, from the smallest (a button on a website) to complex ones (submitting a registration form). You may use them once or consistently, and they consist of one or several parts. In any case, they are individual program parts that, during component testing, are examined separately without interacting with each other. What components of a digital solution are,
We’ve clarified and their testing relatively to Cypress example:
👉 Imagine you need to verify whether a specific button on a website responds to clicks – that is, how users interact with it. Conducting exhaustive E2E testing or any other comprehensive testing for the entire application would be unjustifiably time-consuming and costly. That’s why no QA team can do without it in their daily practice!
Explore topic, it’s tailored just for you:
In such situations, component testing comes to the rescue. You can quickly check the functionality of a specific component. This is the simplest example of a situation where it is reasonable to test components. So, delve into the advantages of component testing in more detail.
Advantages of Isolating and Testing Components
Every QA team that conducts component testing during the development of a web application gains the following advantages:
- Early Defect Detection in SDLC. The quality assurance team can start component testing without waiting to complete the entire application development, thereby identifying defects at the beginning of the workflow. This significantly reduces the cost of bug fixes and saves a substantial amount of project time.
- Enhanced Reliability of the Software Product. Since the functionality and performance of each component are assessed throughout the development process, it increases the likelihood of the overall system’s reliable operation.
- Reuse of Component Code. Confidence in the high quality of each individual component allows the team to use it again in the development of the digital solution, positively impacting development speed.
- Reuse of Test Cases on Project. Additionally, component testing enables the reuse of test environment settings and automation test scenarios when running subsequent component tests. This capability reduces testing time.
- Accessibility of Component Testing Regardless of Development Stage. If the QA team needs to check the operation of a specific component, the functionality of which partially depends on other components that haven’t been created yet, this issue is solvable. In component testing, using mocks and drivers to simulate the interface between components is permissible.
A component test can be executed using various testing tools, but in this material, we want to focus on Cypress component testing, providing Cypress component tests for React applications as an example. Both in detail are covered in the official Cypress documentation. By the way, Cypress docs is cool.
Let’s discuss what React components are and the benefits developers and QA team gain by testing them with Cypress. A React component is an autonomous, reusable code fragment that allows dividing the UI into several independent parts, considering them separately.
Benefits of Cypress for React App Component Testing
Cypress supports component testing for React applications out-of-the-box. It is compatible with multiple frameworks, including Next.js, React with Vite, and React with Webpack.
To start component testing in React using Cypress, of course you need available React project. In our case it is a Vite + React project. According to trends, this is the most popular and recommended way to create React app, unlike those build tool that based on outdated Webpack manager. Since nowadays the development policy of React framework relies on the server side. Therefore, this moments should also be taken into account during testing.
Thus, to showcase key concepts of component testing type, we created a basic project. Although you can find in it the essentials like a counter button and multiple links designed. These web elements provide a concise yet comprehensive demonstration of main component testing principles.
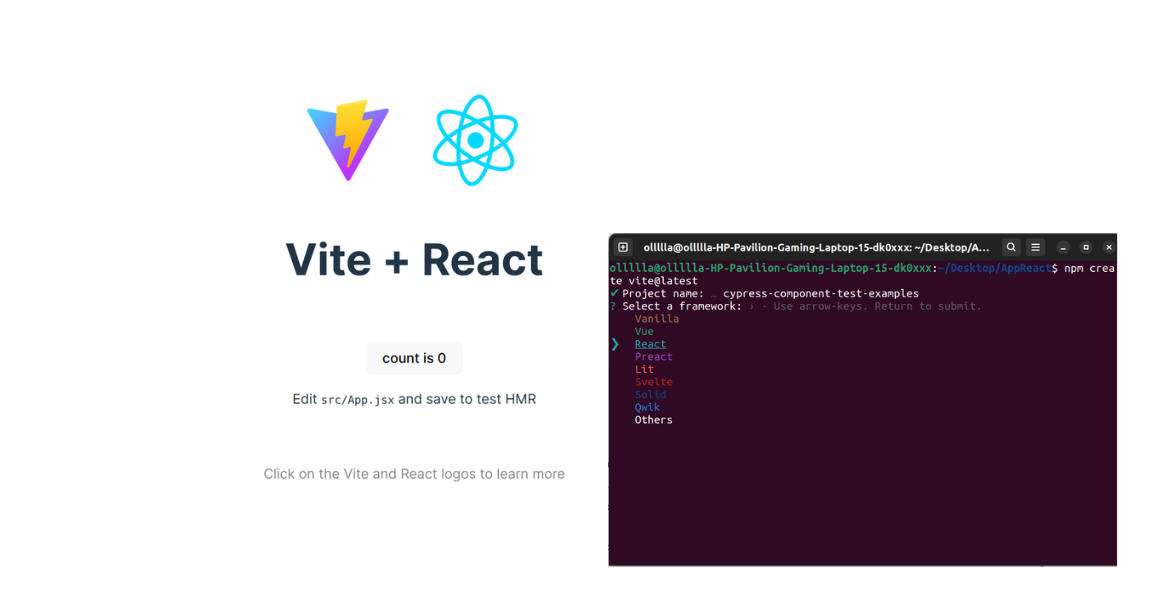
Next follow a few steps:
-
- Install the Cypress testing tool in project folder, for instance using npm:
npm install cypress --save-dev
- Launch Cypress Cloud using the following command:
npx cypress open
- In the opened window, choose the desired testing type; in our case, choose component testing:
Cypress Component Testing Menu - Configure the project using Cypress Launchpad. During setup, your bundler and framework will be automatically detected.
How to pick React Framework Cypress Component testing - Verify the installation of all the required dependencies. It depend from framework. For Vite React build tool with a command:
npm install -D vite
If everything is fine, there will be a green checkmark next to each of them. Even if that’s trouble happens, carefully inspect the console. You can find there an instructions on fixing the issue effortlessly. I did it with of the vs of the following commands, learn more about them on LinkedIn post and its discussion.
npm install --legacy-peer-deps npm install --force
-
Wait for Cypress to generate and add the configuration files to your project.
Cypress Component Testing configuration report - In the next opened window, select the preferred browser:
Cypress Browser option
- Install the Cypress testing tool in project folder, for instance using npm:
After completing these steps, you can begin writing your first test and running actual component tests in your spec file.
A basic example of a Cypress component tests for React App may look like:
import App from '../App.jsx'
describe('ComponentName.cy.jsx', () => {
it('mount', () => {
cy.mount(<App/>)
cy.get('button').click()
cy.contains('button', 'count is')
})
it('Test a Message', () => {
cy.mount(<App/>)
cy.get('p.read-the-docs').should("have.text",'Click on the Vite and React logos to learn more')
})
it('Logo', () => {
cy.mount(<App/>)
cy.get('img.logo.react').click()
cy.wait(2000);
})
it('Header', () => {
cy.mount(<App/>)
cy.get('img.logo.react').click()
cy.wait(2000);
cy.url().should('include', 'https://react.dev/');
cy.contains('main h1', 'React').should('be.visible');
cy.get('div.hidden.sm:block').should("have.text",'Help Provide Humanitarian Aid to Ukraine')
})
})
Understanding the Cypress Folder/File Structures:
- Components folder: Contains Cypress component specs
- Fixtures folder: Any data which can be used inside the specs can be placed here
- Support folder: helper functions, utilities, any reusable code, and custom commands can be placed here.
- Support/component.js: Great place to put the global configuration and reusable code
- Support/commands.js: In this file, you can create custom commands or override existing commands
- Cypress.config.js: This file contains runtime configurations such as devServer, framework, bundler, reporter, videos, screenshot options, etc.
- Package.json: This file tracks all installed dependencies and allows you to create a custom commands shortcut
🎉 Hurray, the result we got!
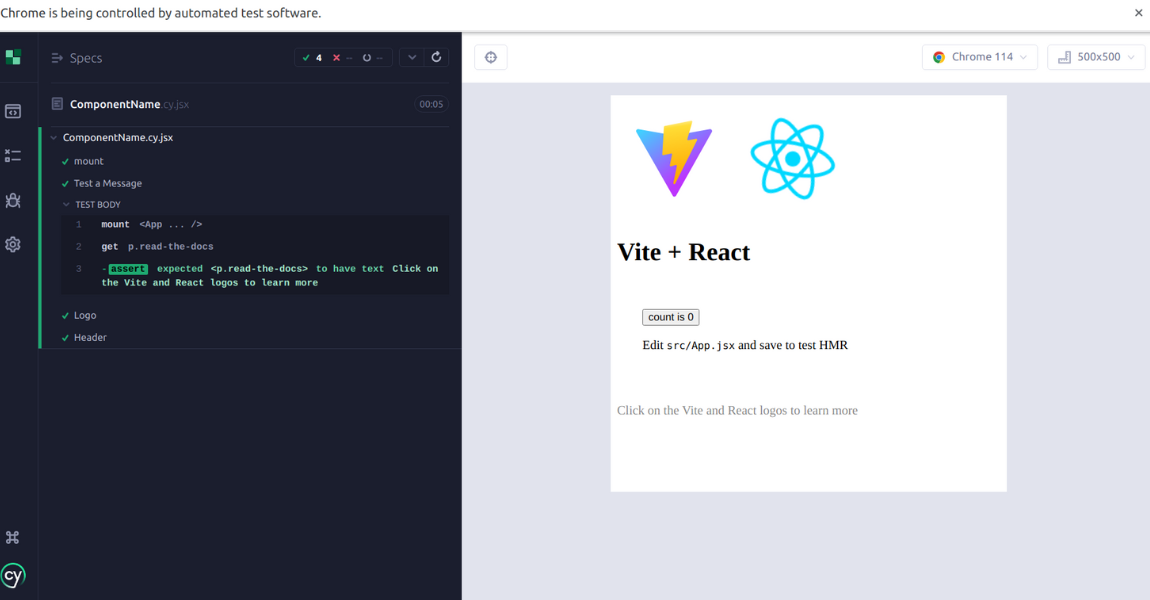
🛠️ Handy CLI commands of Cypress Component Testing
npx cypress run --component //Execute component Tests in headless mode.
npx cypress run --component --config video=false //use the command to to define the Cypress configuration.
npx cypress run --component --headed //Execute Cypress component tests, directly in the headed mode without manually selecting the test files, browser and tests on the test runner window.
npx cypress open --component --browser chrome //Open the test runner window directly by skipping the browser option.
npx cypress run --component --spec cypress/component/ComponentName.cy.jsx
Follow this link to know how to Run Cypress Component tests alternatively:
The Ultimate Cypress Tutorial: How to Organize your Advanced Testing Framework
Key Features of Cypress Component Testing
If you’re in the process of selecting a test framework for conducting component testing for your application, you should consider Cypress for the following unique features in the QA process:
- Snapshot Capabilities. Cypress takes snapshots during test runs, allowing you to hover over Cypress commands and instantly see what happened at that point in testing.
- Fast, Efficient Debugging of Tests. Understanding why specific tests fail is straightforward in Cypress. The stack traces available in the framework and familiar tools like Developer Tools make debugging tests simple and high-quality.
- Automatic Waiting. The tool defaults to executing a command only when the page elements are ready. There’s no need to add sleep or wait statements to your component tests.
- Stubs, Spies, and Clocks. Cypress provides commands like
cy.stub()
,cy.spy()
, andcy.clock()
to control the behavior of functions and time intervals in your application. - Network Traffic Stubbing. You can test edge cases without involving your server.
- Reliable Testing Results. Cypress’s architecture doesn’t rely on Selenium WebDriver, ensuring you get reliable and fast component tests.
- Visualization of Test Results. The framework allows you to view screenshots and video recordings of tests, making it easy to identify the cause of failures and resolve them more quickly.
- Unreliable Test Detection. Cypress has a built-in Flaky Test Management System that eliminates tests showing incorrect results.
All these tool capabilities make Cypress component testing fast and reliable, enabling teams to release high-quality software products in a short timeframe.
Advanced Cypress Component Testing Techniques
Cypress is a versatile web application testing tool that supports various tests, progressive testing approaches, including BDD approach and parallel test execution using cloud services. Let’s delve into advanced techniques that make component testing with Cypress even more effective.
Cross-browser Testing
You can run component tests in multiple browsers: Chrome, Electron, Microsoft Edge, Safari, and Firefox. You can find the full list of available browsers in the Cypress menu.
Specify the browser for testing using the –browser flag or a specific command. For instance, the command would look like this for Firefox:
cypress run --browser firefox
For added convenience, use scripts:
Note: To run tests on a CI pipeline, its containers must be installed locally or in the cloud. We’ll further discuss running Cypress in continuous integration mode.
Parallel Testing
Cypress supports parallel test execution without additional requirements. To parallelize a test suite, simply use the following command when running tests in CI:
cypress run --record --parallel
Intelligent Orchestration
After setting up recording in Cypress Cloud, additional capabilities become available. For instance, you can rerun failed specs using Spec Prioritization and cancel test runs with Auto Cancellation in case of unexpected failures.
Recording Tests in Cypress Cloud
Cypress users can access the Cypress Cloud web component, providing detailed testing results. Here, you can view videos and screenshots, check the ratio of skipped, passed, failed, and pending tests, inspect the stack trace, and more.
Summarizing
Component tests are a crucial component of the software development life cycle. A quality assurance team can ensure that each individual component of a digital solution works as expected. This, in turn, contributes to the creation of higher-quality and more reliable products that working properly.
Such tests can be executed in various frameworks, also an actively utilized tool for web application component testing is Cypress. It supports parallel and cross-browser testing, allowing tests to be run locally and in CI mode.
If you have any questions regarding component testing or any other types of testing, including their automation, feel free to reach out to the testomat.io team. We are ready to address each inquiry and make your QA processes more effective and streamlined.