JavaScript is a popular programming language commonly used for web development. According to the StackOverflow Survey of 2023, it has been the top-used programming language for 10 years. TypeScript places the fifth position. QA engineers might have heard about TypeScript. Now it’s not just for developers anymore – QA teams also widely use it with automation testing to catch errors before they even happen saving them tons of time.
Why TypeScript for Software Testing?
TypeScript promotes clear code, simplifies collaboration across QA and development teams, and makes the testing process faster. It also helps them keep their tests reliable and maintainable as the projects grow.
⬇️ Here we are going to overview why testers should pay attention to the rising popularity of TypeScript and ideally start using it.
What’s exactly Typescript?
When extending JavaScript, Typescript adds a key feature – static typing! Unlike JavaScript, where variables can change their data type on the fly, TypeScript allows teams to define data types for variables, functions, and other elements, to provide more structure, and help detect bugs early in the development process. In addition to that, when adopting test-driven development (TDD) alongside TypeScript, QA engineers can write tests inside the development process itself and make them not only functional but also easily testable. Thus, TypeScript combined with a test-driven development (TDD) approach allows teams:
- To drive design decisions to make sure that test cases clearly define expected behaviors
- To establish the high-level organization of the code
- To write maintainable and testable code
Key Features and Advantages of Typescript Testing
TypeScript offers a wide range of advantages for testing and provides QA teams with test frameworks to improve code quality and reliability. When utilizing TypeScript’s features, teams can minimize downstream issues early and keep test cases clear and readable.
- Static Typing. By defining variable and function types, TypeScript enables teams to identify errors and anomalies during development, even before test scripts run. This significantly reduces debugging time for QA engineers and minimizes time on fixing errors in code.
- Clear Code. Type definitions function as inline documentation that improves test code readability. Everyone in the team can quickly understand the logic and purpose of test scripts leading to effortless and productive collaboration and reducung time spent on analyzing complex code. Furthermore, clear type definitions make maintaining large test suites more manageable as the project grows.
- Confident Refactoring. With TypeScript, teams can modify test code with greater confidence. Type checks will prevent their modifications from breaking existing functionalities and refactor test code confidently. These checks guarantee that the test suite stays functional.
- Scalability. Clear type definitions become even more important as projects grow. They help keep everything consistent, prevent bugs caused by data types and make large codebases easier to understand and manage.
Reasons why Automation QAs choose Typescript
Here’s a breakdown of why Quality Assurance (QA) engineers are increasingly turning to TypeScript for writing test automation scripts:
- Teams can define data types for their test code and make tests more isolate and stable.
- With TypeScript static typing, teams can detect errors during development, not just execution.
- When making changes, QAs don’t break existing functionality and can refactor test cases with confidence.
- Teams can manage and understand large test suites in terms of TypeScript’s code clarity and consistency.
Popular testing strategies with TypeScript
To carry out effective TypeScript testing, QA engineers can use a combination of strategies. Let’s overview them in detail below:
Unit Testing
Typescript unit testing is about evaluating individual units or components of your code separately from the rest. To achieve this isolation, mocks and stubs are frequently utilized to replace dependencies. This approach guarantees that each unit operates correctly and meets its design specifications.
By focusing on isolated components, Typescript unit testing facilitates the early detection of bugs and simplifies debugging. It also provides a stable base for code refactoring without breaking existing features.
How to perform TypeScript unit testing with Jest
Let’s discover how to write your first unit test in TypeScript with Jest:
You should initialize your project by creating a new test folder, for instance, name it Jest_Demo:
mkdir Jest_Demo
cd Jest_Demo
mkdir src
mkdir test
Next you should set up a package.json
file to manage your project’s dependencies and scripts. Also, you should install Jest, TypeScript, and necessary type definitions (ts-jest and @types/jest) to integrate testing with type safety.
npm init -y
npm i -D typescript jest ts-jest @types/jest
At this step, you need to create a tsconfig.json
file to specify TypeScript compiler options, such as the target JavaScript version, module system, and directories to include. This configuration guarantees that your TypeScript code compiles correctly according to your project’s needs.
npx tsc --init
Check
{
"compilerOptions": {
"target": "es2016",
"module": "commonjs",
"esModuleInterop": true,
"forceConsistentCasingInFileNames": true,
"strict": true,
"skipLibCheck": true,
"rootDir": "./src",
"outDir": "./dist",
"sourceMap": true
}
}
You need create a jest.config.js
file Also, you should configure it to use ts-jest to handle TypeScript files, define the test environment (Node.js), and specify the test file pattern.
npx ts-jest config:init
Here it is imperative to organize your project with a src folder for your TypeScript files.
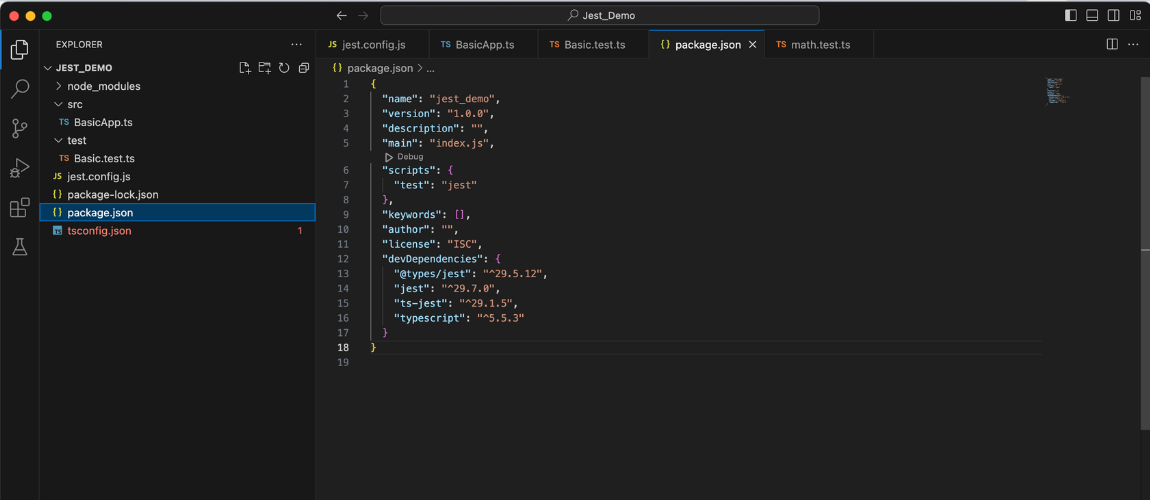
You need to write your first Jest TypeScript test, for such a simple App to paste into a BasicApp.ts file:
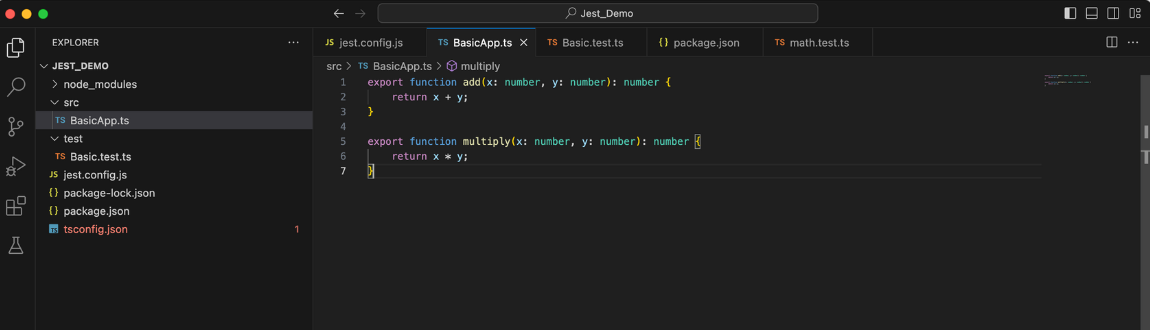
with a Basic.test.ts The following example:
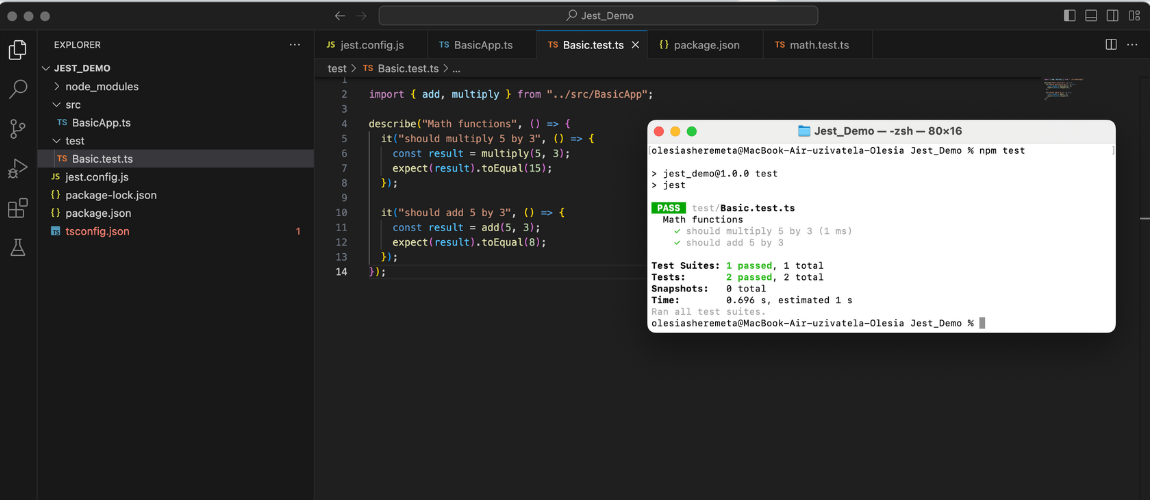
End-to-End Testing
End-to-end (E2E) testing replicates real user interactions and scenarios with the application. It validates the complete application workflow and is especially effective at identifying problems that may occur from the integration of various components. By simulating actual user behavior, E2E tests confirm that all parts of the system function effectively.
This testing method is essential for detecting and addressing issues that could affect the user experience and guarantees that the application performs correctly under real-world conditions.
How to write E2E tests in TypeScript Playwright
Let’s overview how to write tests in TypeScript with Playwright:
You must ensure that Node.js and npm are installed on your system.
node -v
npm -v
Once you have Node.js and npm set up, you can create your project folder. After then using the following command install Playwright:
npm install playwright@latest
Playwright testing framework offers support for JavaScript and TypeScript languages. In our case choose the last to initialize and set up the basic structure for working with TypeScript code. Also, the installer asks you for a path where you put your tests, to add GitHub Actions workflow and install Playwright browsers. Playwright supports multiple browsers: Chromium, Firefox, and WebKit.

So, your test will be saved in the test folder into spec.ts
file. Here is a tree structure of the Playwright Demo project:
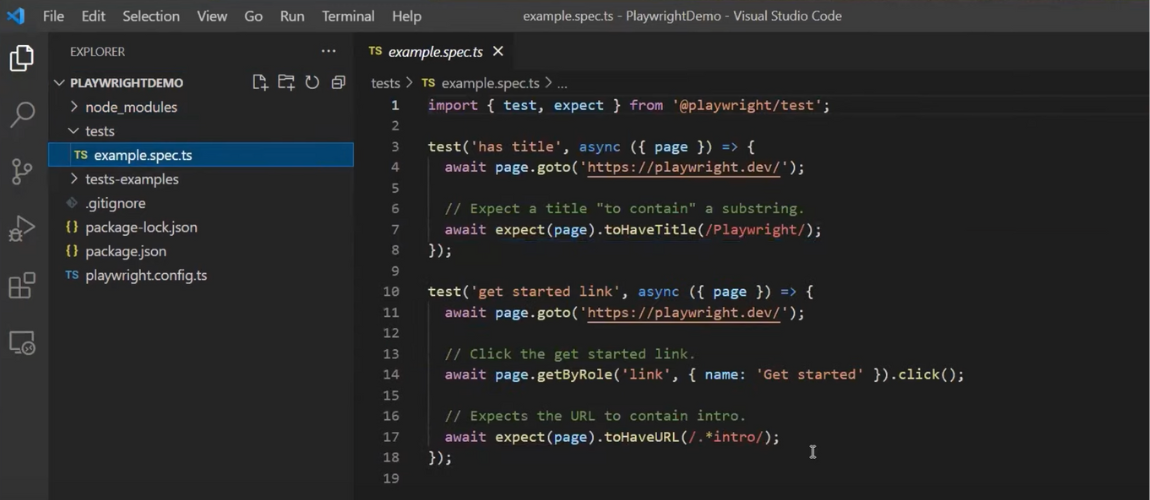
Pay attention, the page
is an object name that might be named any as you want.
You may run the tests using the following command:
npx playwright test
If you need to choose the browser you want to use by adding the --browser
flag when running your tests. Here are the examples:
npx playwright test --browser=chromium # For Chromium
npx playwright test --browser=firefox # For Firefox
npx playwright test --browser=webkit # For WebKit
This is how we launched our first Playwright TypeScrit project and executed our first test.
Follow the next links if you are interested in Plawright automation:
- 💫 Journey: Migrating from Selenium to Playwright
- What is the use of fixtures in Playwright?
- How to Organize an Advanced (Scaled) (e2e/unit) Playwright Testing Framework
- Grouping Playwright Tests for Improved Framework Efficiency
- Debugging & Tracing Playwright features – tips, techniques to running & debugging your tests 🔎
Best Practices in TypeScript for QA Engineers
Here we are going to explore the best practices for QA engineers who want to use TypeScript’s strengths to make their testing process streamlined and write more reliable test cases.
✅ Use Arrange-Act-Assert (AAA) Pattern in TypeScript
The Arrange-Act-Assert (AAA) pattern is a widely adopted best practice for structuring unit tests. This pattern helps create clear, understandable, and maintainable unit tests by dividing the code of the test cases into three distinct sections: Arrange, Act, and Assert. Here is how it works in the context of TypeScript:
- Arrange: Set up the necessary conditions and inputs for the test cases.
- Act: Execute the function or method under test.
- Assert: Verify that the output is as expected.
✅ Consistent Code Style
Writing code consistently makes it clear for everyone on the team. This helps with teamwork because teammates can understand each other’s code easily. It also makes it simpler to fix and update the code later. Some tools can automatically format your code and check it against common best practices as well as improve code quality and make collaboration productive.
✅ Catch Errors
Writing code that can handle errors is key to building strong and dependable TypeScript applications. Techniques like try…catch blocks let developers manage unexpected issues and give helpful error messages. They can also create custom error classes to hold specific error details, making it easier to deal with different error situations consistently.
By using these methods, they can make sure the applications can bounce back from errors without problems and keep working as expected. Strong error handling also makes debugging easier and helps keep the code quality high.
✅ Type Declarations
Using type declarations and interfaces in TypeScript helps define the kind of data your objects and functions to improve code clarity and type safety. They guarantee that code adheres to expected structures and behaviors. Managing third-party type definitions is made easier with DefinitelyTyped, a repository of high-quality TypeScript type definitions for popular libraries. This allows seamless integration and type safety for third-party packages and delivers error-free development experience. Leveraging type declarations and interfaces significantly improves maintainability and collaboration within a project.
✅ Keep Your TypeScript Code Clear with Code Documentation
Documenting TypeScript code effectively is crucial for maintaining clarity and ease of understanding within a codebase. Using different testing tools, developers can annotate functions, classes, and modules to describe their purposes, parameters, and return types as well as automate the generation of comprehensive and well-structured documentation from these comments. With proper documentation in hand, they can onboard new team members quickly and reduce the time spent on understanding and interpreting code.
Best Tools for QA Engineer
If your QA teams work with TypeScript, they should choose the right tools to carry out efficient testing and maintain code quality. Fortunately, TypeScript can be integrated with many popular testing frameworks and libraries to support type safety and modern development practices.
When performing unit testing, QAs can take into account test frameworks like Jest. With a focus on end-to-end testing, teams can use the Playwright test framework to simulate real user interactions with the application. Combined with TypeScript plugins, ESLint helps teams maintain a consistent code style and catch potential anomalies and issues early.
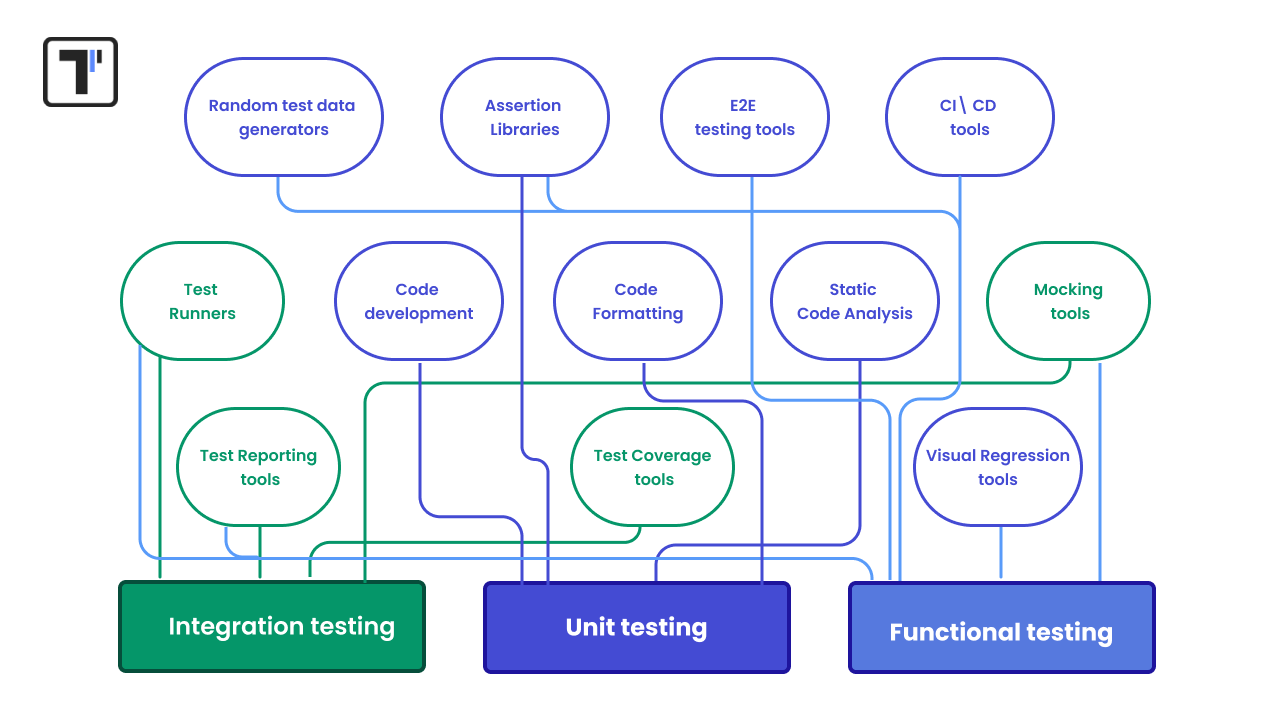
Tools such as Prettier enable QAs to keep code style consistent, minimizing style-based bugs. Furthermore, when integrating Continuous Integration (CI) tools like CircleCI into the workflow allows teams to automate the testing process, provide configurable pipelines to run tests, drive code quality, and deploy applications quickly.
Below you can find a table that provides a comprehensive overview of the best tools available for QA engineers who work with TypeScript. It covers various aspects of testing, code quality, and documentation:
Tool | Purpose | Key Benefits |
![]() |
Unit Testing | Writing clear unit tests, providing fast execution, and features like mocking |
![]() |
E2E Testing | Running automated tests across browsers, writing in TypeScript for type safety E2E Testing |
![]() |
Unit Testing | Providing potentially faster, experimental UI component testing (similar to Jest) |
![]() |
E2E Testing | Offering a visual editor or TypeScript for tests, time travel debugging, and waits for elements |
![]() |
Code development | Powerful and efficient editor for TypeScript development. Provides smart code completions, real-time type error detection, debugging and refactoring. Provides a rich ecosystem of extensions that enhances functionality specific to TypeScript development. |
![]() |
Static Code Analysis | Applying code style, catching errors early, improving code quality |
![]() |
Static Code Analysis | Identifies code smells, bugs, and security vulnerabilities early in development, allowing teams to improve their codebase. |
![]() |
Static Code Analysis | It is an extension for VSCode. Allows developers to identify and report errors early by warnings, codebase stays healthy always thereby apparently fixing ones. |
![]() |
Documentation Generation | Generating API docs, and improving project understanding for QA software engineers |
![]() |
Code Formatting | Providing consistent formatting, saving time, and improving readability |
![]() |
Continuous Integration | Automated testing and deployment, faster feedback, earlier issues identification |
![]() |
Unit Testing (with Chai) | A popular choice, working with TypeScript and Chai assertions |
![]() |
Assertions (with Mocha) | Providing BDD-style assertions for tests written in Mocha. |
Bottom Line: Ready to improve your QA process?
For QA engineers seeking to streamline their testing process, TypeScript offers a great solution. By thoroughly testing software products, QA teams can not only identify errors during development but also write more reliable test scripts. If combined with clear code practices and type definitions, they can create maintainable and scalable test suites that contribute to the development of high-quality TypeScript apps with more effective testing activities.
📍 Would you like your tool integrated with TypeScript to be listed in our catalogue?
Also drop us a line to learn more about how TypeScript can improve your QA workflow.
👉 Contact Us to learn more about details!